How to show content
January 30, 2024About 4 min
How to show content
- A web application consists of content:
- Titles
- Images
- Paragraphs
- Forms
- Lists
- ...
- An Angular application is a collection of
components
- An Angular application is a single page application (SPA)
- Each
component
is a part of the web page
Article
- The main purpose of our news app is to show articles to visitors
- Such an article is build with
HTML
andCSS
and may contain- A title
- A subtitle
- The body/content
- ...
- In Angular this article will be a
component
Article component
- Create the article component by executing the following command in the
src/app
folder:
ng g c article
ng g c article
ng
: we're making use of theAngular CLI
g
: shorthand notation forgenerate
c
: shorthand notation forcomponent
article
: name of thecomponent
component name with multiple words
- When generating a component with a name with multiple words you should separate these words with a dash (
-
) - Like:
ng g c article-detail
- Generating the article component results in a new
article
folder in thesrc/app
folder containing all the files needed for an Angular component:
file | description |
---|---|
article.component.html | HTML that will be rendered when we call the component (required) |
article.component.css | CSS that is used to style this component (required) |
article.component.ts | Code behind this component (required) |
article.component.spec.ts | Unit tests for this component (optional) |
article.component.html
- Contains the
HTML
that will be rendered. - Works together with
article.component.ts
- For now we just write the
HTML
for our article
<article>
<h1>Biden for president</h1>
<h4>A crushing win over D. Trump</h4>
<figure>
<img src="https://images.pexels.com/photos/1202723/pexels-photo-1202723.jpeg?auto=compress&cs=tinysrgb&dpr=2&h=100&w=134"
alt="flag America">
<figcaption>Image source: Pexels</figcaption>
</figure>
<p>
Lorem ipsum dolor sit amet consectetur adipisicing elit. Tenetur voluptas sequi voluptatum pariatur! Quae cumque
quidem dolor maxime enim debitis omnis nemo facilis sequi autem? Quae tenetur, repellat vero deleniti vitae
dolores? Cum tempore, mollitia provident placeat fugit earum, sint, quae iusto optio ea officiis consectetur sit
necessitatibus itaque explicabo?
</p>
<p>Lorem ipsum dolor sit amet consectetur adipisicing elit. Tenetur voluptas sequi voluptatum pariatur! Quae cumque
quidem dolor maxime enim debitis omnis nemo facilis sequi autem? Quae tenetur, repellat vero deleniti vitae
dolores? Cum tempore, mollitia provident placeat fugit earum, sint, quae iusto optio ea officiis consectetur sit
necessitatibus itaque explicabo?</p>
<footer>
<p>Author: Michaƫl Cloots, Published: 28/11/2020</p>
</footer>
</article>
article.component.css
- Next we add some basic styling for our article.
- We do this in the
article.component.css
:
article {
border: 2px solid lightgrey;
box-shadow: 2px 2px 2px lightgrey;
padding: 10px;
min-width:250px;
max-width:600px;
margin: 10px auto;
}
img {
max-width: 100%;
height:auto;
}
figure {
text-align: center;
}
figcaption {
font-style:italic;
text-align: center;
}
article p {
text-align: justify;
}
article footer {
font-style:italic;
font-variant:small-caps;
}
Tailwind CSS
- Later on we will integrate Tailwind CSS in our project!
article.component.ts
- This code file is the class file of the
component
- Showing content on your web application is nothing more than calling (or routing to)
components
- These
components
areclasses
that will be initialized - The rendering process returns
HTML & CSS
to the application, which will be shown on the place where we called thecomponent
- The
ArticleComponent
class file looks as follows:
import { Component } from '@angular/core';
@Component({
selector: 'app-article',
standalone: true,
imports: [],
templateUrl: './article.component.html',
styleUrls: ['./article.component.css']
})
export class ArticleComponent {
}
code | description |
---|---|
import { Component } from '@angular/core'; | JavaScript import that is needed for working with components |
@Component { ... } | Decorator function that specifies the Angular metadata for the component . This tells Angular that the ArticleComponent class is a component |
selector: 'app-article' | Element selector for the component . It matches the name of the HTML element that identifies this component. In this case <app-article></app-article> |
standalone: true | Specifies this is a standalone component |
templateUrl: './article.component.html' | The location of the component's template file = HTML |
styleUrls: ['./article.component.css'] | The location of the component's private CSS styles = CSS |
export class ArticleComponent implements OnInit { | Class definition for ArticleComponent . Always export the component class so you can import it elsewhere where you need it |
constructor() {} | Constructor for this class |
Class file
- For now we don't have to code in this class file
- Later on we will have to add code because:
- Some business logic needs to be applied
- We need to communicate with our
API
to read and post data - ...
Showing our article
- By default an Angular application consists of 1 component =
AppComponent
- In the
main.ts
file you can see that theAppComponent
is loaded when the web application is bootstrapped:bootstrapApplication(AppComponent, appConfig).catch((err) => console.error(err));
- In the
app.component.ts
file you can see that theapp.component.html
file is rendered when thiscomponent
is initialized:templateUrl: './app.component.html'
- The
app.component.html
is the starting/home page of our single page web application - Remove all the code and add our recently created
ArticleComponent
to show our article
<app-article></app-article>
- Notice that this gives us an error (NG8001) that you will likely see a lot while developing Angular applications: 'app-article' is not a known element
- This error occurs because our
AppComponent
doesn't know anything about theArticleComponent
. For this reason we have to import ourArticleComponent
by adding it to theimports
array inapp.component.ts
:
//app.component.ts
import { Component } from '@angular/core';
import { RouterOutlet } from '@angular/router';
import { ArticleComponent } from "./article/article.component";
@Component({
selector: 'app-root',
standalone: true,
imports: [RouterOutlet, ArticleComponent],
templateUrl: './app.component.html',
styleUrl: './app.component.css'
})
export class AppComponent {
title = 'my-news';
}
Result:
- You can show the result by running the command
npm start
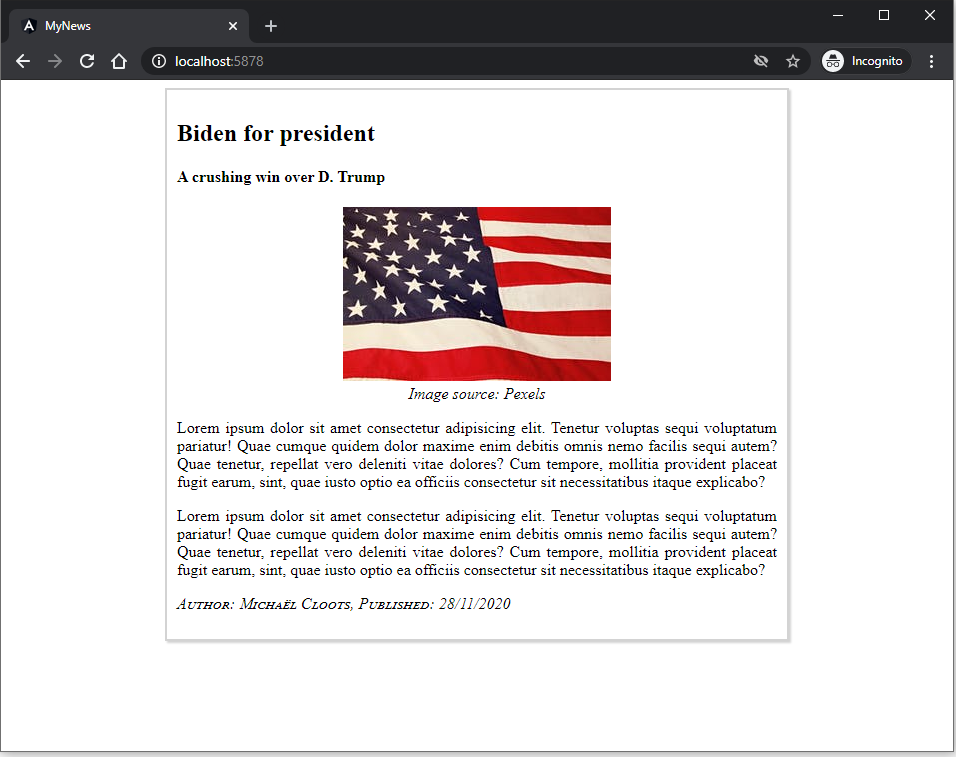